Optimize Your PHP Code: 8 Functions You Need for Efficient Table Handling
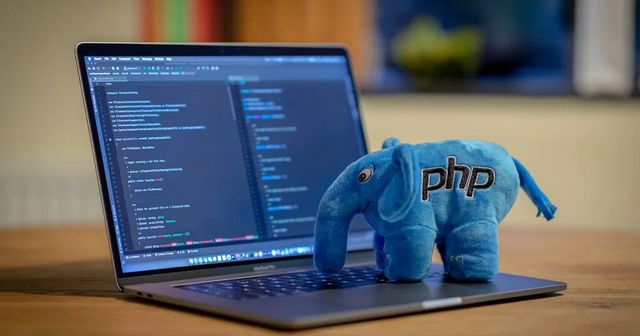
If you want to become a good PHP developer, you must learn to work with arrays. Arrays are used a lot in PHP: temporarily store, organize, and process data before it's saved in a database. Knowing how to work with them efficiently will help you manage and process data more effectively.
In this article, we'll look at eight important functions for working efficiently with arrays in PHP. These functions will help you improve your code and solve difficult problems more easily.
1. array_map
The array_map
function applies a given function to each element of one or more arrays and returns an array containing the results. Note that the keys of the original arrays are not affected: they are retained in the resulting array, even if the values are modified.
Example:
$numbers = [1, 2, 3, 4];
$squaredNumbers = array_map(function($n) {
return $n * $n;
}, $numbers);
print_r($squaredNumbers);
// Résultat : [1, 4, 9, 16]
💡 Tip: If you don't need to create a new array, but simply want to modify the existing one, you can use array_walk
. array_walk
applies a function to each element of the array but modifies the array directly, without returning it. This can be more efficient in some cases.
Example with array_walk
:
$numbers = [1, 2, 3, 4];
array_walk($numbers, function(&$n) {
$n *= $n;
});
print_r($numbers);
// Résultat : [1, 4, 9, 16]
As you can see, 'array_walk' directly changes the $numbers array without making a new one.
2. array_reduce
The array_reduce
function is used to find a single total by applying a callback function to each element of an array. The "value" that it produces can be of any type, including array, string, or object. The type of value it produces depends on the logic defined in the callback
Example:
$numbers = [1, 2, 3, 4];
$sum = array_reduce($numbers, function($carry, $item) {
. return $carry + $item;
}, 0);
echo $sum;
// Résultat : 10
3. array_filter
The array_filter
function filters the elements of an array based on a callback function. Only the elements that return true will be included in the returned array. The callback function can also accept the key of each element. This allows filtering not only by value but also by key.
If you need more information, check the official documentation on array_filter with mode.
Example:
$numbers = [1, 2, 3, 4, 5];
$evenNumbers = array_filter($numbers, function($n) {
. return $n % 2 === 0;
});
print_r($evenNumbers);
// Résultat : [2, 4]
4. array_merge
The array_merge
function combines one or more arrays into a single one. This function is used to merge several data sets.
Example:
$array1 = ["a" => "pomme", "b" => "banane"];
$array2 = ["a" => "ananas", "c" => "citron"];
$result = array_merge($array1, $array2);
print_r($result);
// Résultat : ["a" => "ananas", "b" => "banane", "c" => "citron"]
5. array_keys
The array_keys
function returns all the keys of an array or the keys that correspond to a specified value. It's particularly useful for getting the indices from associative arrays.
Example:
$array = ["a" => "pomme", "b" => "banane", "c" => "citron"];
$keys = array_keys($array);
print_r($keys);
// Résultat : ["a", "b", "c"]
However, to check whether a key exists in an array, it's better to use array_key_exists
than to get all the keys with array_keys
and then use in_array
. Using array_key_exists
is more direct and avoids unnecessary steps 🚀
$array = ['nom' => 'Jean', 'âge' => 30];
if (array_key_exists('nom', $array)) {
echo 'La clé "nom" existe dans le tableau';
}
6. array_values
The array_values
function returns all the values in an array. I suggest using it when you want to get an array of numbers from another array.
Example:
$array = ['a' => 'pomme', 'b' => 'banane', 'c' => 'citron'];
$values = array_values($array);
print_r($values);
// Résultat : ['pomme', 'banane', 'citron']
7. array_unique
The array_unique
function gets rid of duplicate values in an array. This function is useful for getting a set of different values.
Example:
$array = ['pomme', 'banane', 'pomme', 'citron'];
$uniqueArray = array_unique($array);
print_r($uniqueArray);
// Résultat : ['pomme', 'banane', 'citron']
8. array_combine
The array_combine
function creates an array by combining two different arrays. One array has the keys, and the other has the values. Each key in the first array will be linked to a value in the second array. This makes it useful for combining two sets of data, like names and ages.
Example:
$keys = ['nom', 'prénom', 'âge'];
$values = ['Dupont', 'Jean', 30];
$resultat = array_combine($keys, $values);
print_r($resultat);
// Résultat :
// Array
// (
// [nom] => Dupont
// [prénom] => Jean
// [âge] => 30
// )
Conclusion
Knowing how to change arrays is a basic skill for any PHP developer. If you learn these functions, you'll be able to work with data more efficiently and write cleaner, more maintainable code. Whether you're transforming, filtering, merging, or reducing arrays, these tools are essential for solving the day-to-day problems you'll face in your projects. Take the time to understand how they work and add them to the tools you use every day.
You can find more information about array functions and learn more about PHP on the official documentation website: https://www.php.net/manual/en/ref.array.php. There, you'll find a list of all the functions available for working with arrays, along with examples and explanations.